Google Ads Keyword Planner API는 Google Ads의 키워드 계획 및 연구 도구를 프로그래밍 방식으로 활용할 수 있게 해주는 API입니다. 이를 통해 키워드 아이디어, 검색량, 경쟁 수준 등을 API로 받아올 수 있습니다. Google Ads Keyword Planner API는 블로그 콘텐츠 전략 수립과 SEO 최적화에 매우 유용하게 활용할 수 있습니다. 자세한 내용은 아래 블로그를 참고 바랍니다.
Google Ads Keyword Planner API 활용한 데이터 기반 블로그 콘텐츠 전략 수립과 SEO 최적화
Google Ads Keyword Planner API 활용한 데이터 기반 블로그 콘텐츠 전략 수립과 SEO 최적화
Google Ads Keyword Planner API는 블로그 콘텐츠 전략 수립과 SEO 최적화에 강력하게 활용할 수 있는 도구입니다.이를 통해 키워드 데이터를 효과적으로 수집하고 분석하여 콘텐츠 아이디어 발굴, 트렌
gsixplus.tistory.com
이제 본격적으로 구글 키워드 애즈 API 코드를 살펴보겠습니다.
필요한 파일은 크게 google_keyword_planner.py와 google-ads.yaml 두 개입니다.
이 두 개의 파일만 있으면 구현 가능합니다.
다만 실행에 있어서 필요한 토큰과 코드를 발행하는 것이 다소 복잡할 수 있습니다.
하지만 아래 내용과 추가 블로그에 링크된 실제 구현 블로그 글을 따라하시면 무난히 구현이 가능합니다.
시작해볼까요?
시작에 앞서 알아야 될것
필요한 환경: Python 및 pip (Python 3.11.4)
- 필요한 파일: google_keyword_planner.py, google-ads.yaml ( 이 두 개의 파일만 있으면 구글 키워드 플래너 API 구현이 가능합니다.)
- keyword planner 실행 방법: python google_keyword_planner.py
- refresh token 발행 실행 방법: python generate-refresh-token.py
- pip install google-ads , pip install google-auth google-auth-oauthlib google-auth-httplib2
1. 코드
google_keyword_planner.py
from google.ads.googleads.client import GoogleAdsClient
from google.ads.googleads.errors import GoogleAdsException
import sys
def get_keyword_ideas(customer_id, location_ids, language_id, keyword_texts):
client = GoogleAdsClient.load_from_storage("YOUR_PATH_TO/google-ads.yaml")
keyword_plan_idea_service = client.get_service("KeywordPlanIdeaService")
# 요청 객체 생성
request = client.get_type("GenerateKeywordIdeasRequest")
request.customer_id = customer_id
# 언어 설정
request.language = f"languageConstants/{language_id}"
# 위치 설정
request.geo_target_constants = [
client.get_service("GeoTargetConstantService").geo_target_constant_path(location_id)
for location_id in location_ids
]
# 키워드 네트워크 설정
request.keyword_plan_network = client.enums.KeywordPlanNetworkEnum.GOOGLE_SEARCH_AND_PARTNERS
# 키워드 텍스트 추가
request.keyword_seed.keywords.extend(keyword_texts)
try:
# 요청 실행
keyword_ideas = keyword_plan_idea_service.generate_keyword_ideas(request=request)
# 결과 출력
for idea in keyword_ideas:
competition_level = client.enums.KeywordPlanCompetitionLevelEnum(
idea.keyword_idea_metrics.competition
).name
print(
f'Keyword: "{idea.text}", '
f"Avg. Monthly Searches: {idea.keyword_idea_metrics.avg_monthly_searches}, "
f"Competition: {competition_level}"
)
except GoogleAdsException as ex:
print(f"Request failed with status {ex.error.code().name} and includes the following errors:")
for error in ex.failure.errors:
print(f"\t{error.error_code}: {error.message}")
sys.exit(1)
if __name__ == "__main__":
customer_id = "YOUR_TEST_ACCOUNT_CUSTOMER_ID"
location_ids = ["2410"] # 한국 코드
language_id = "1012" # 한국어 코드
keyword_texts = ["행복", "돈", "아름다움"]
get_keyword_ideas(customer_id, location_ids, language_id, keyword_texts)
- google_keyword_planner.py코드 수정이 필요한 부분
"YOUR_PATH_TO/google-ads.yaml"
예시:"D:/googleTrendsAPI/google-ads.yaml"
"YOUR_TEST_ACCOUNT_CUSTOMER_ID"
예시:2381517421
2. yaml 파일
google-ads.yaml
google-ads.yaml 파일의 예시는 다음과 같습니다:
developer_token: YOUR_DEVELOPER_TOKEN
client_id: YOUR_CLIENT_ID
client_secret: YOUR_CLIENT_SECRET
login_customer_id: YOUR_LOGIN_CUSTOMER_ID
use_proto_plus: True
refresh_token: YOUR_REFRESH_TOKEN
google-ads.yaml에 값 발행 방법
- developer_token: Google Ads 계정 생성 페이지에서 개발자 토큰을 발행받을 수 있습니다.
- client_id 및 client_secret: Google Cloud Platform API 자격 증명 페이지에서 프로젝트 생성 후 "OAuth2 클라이언트 ID"를 생성하여 발급받습니다.
- login_customer_id: Google Ads 계정 생성 시 페이지 우측 상단에 표시된 고객 ID를 사용합니다. 코드에서는 하이픈을 제외하고 입력합니다.
- use_proto_plus:
True
는 Google Ads API의 프로토콜 버퍼 데이터를 Python 친화적으로 처리하기 위해 설정됩니다. - refresh_token: 인증 후 로그인에 사용되는 토큰입니다. 아래 제공된 코드를 사용해 발행합니다.
refresh_token 발행 코드
generate-refresh-token.py
import json
from google.auth.transport.requests import Request
from google_auth_oauthlib.flow import InstalledAppFlow
def generate_refresh_token(client_id, client_secret):
flow = InstalledAppFlow.from_client_config(
{
"installed": {
"client_id": client_id,
"client_secret": client_secret,
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://oauth2.googleapis.com/token",
}
},
scopes=["https://www.googleapis.com/auth/adwords"],
)
credentials = flow.run_local_server(port=8080)
print("Access token:", credentials.token)
print("Refresh token:", credentials.refresh_token)
return credentials.refresh_token
if __name__ == "__main__":
client_id = "YOUR_CLIENT_ID"
client_secret = "YOUR_CLIENT_SECRET"
refresh_token = generate_refresh_token(client_id, client_secret)
print(f"Your refresh token: {refresh_token}")
client_id와 client_secret은 Google Cloud Console에서 생성한 것을 입력하여 사용하세요.
이후 실행하면 로그인 요청이 들어오고 이를 수행하기 위해 두 가지 설정이 필요합니다.
사용자 인증 정보 화면에서 OAuth 2.0 클라이언트 ID 수정 페이지에 들어가면 승인된 리디렉션 URI를 볼 수 있습니다. 이를 아래와 같이 8080 포트로 맞춰 줍니다.
승인된 리디렉션 URI 등록
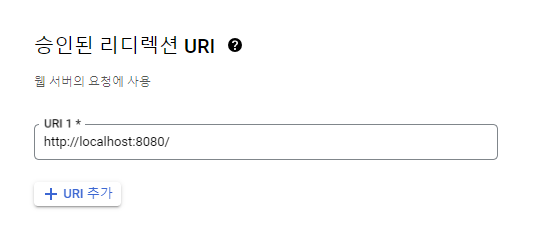
OAuth 동의 화면에서 해당 GCP에 접근할 수 있는 계정을 테스트 사용자로 미리 등록합니다. 구글 계정 이메일을 입력합니다.
테스트 사용자 등록
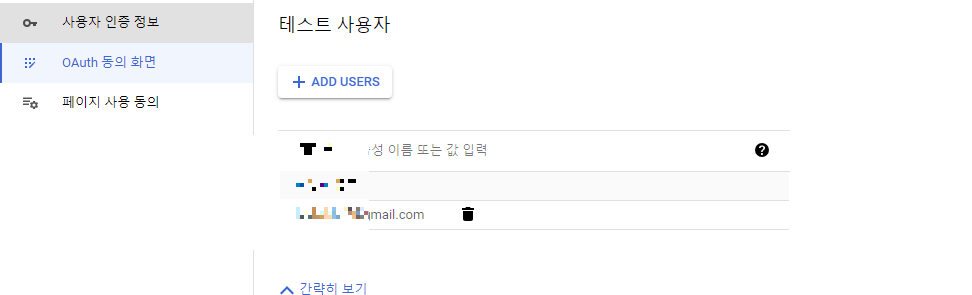
이후 refresh token을 발급 받아서 yaml 파일에 넣어줍니다. python generate-refresh-token.py
명령어를 통해 콘솔 창에서 refresh token을 받을 수 있습니다.
구글 키워드 애즈 API 실제로 구현했을 때 예시
구글 키워드 애즈 API 실제로 구현 했을 때 예시
필요한 환경python 및 pip (Python 3.11.4) 필요한 파일google_keyword_planner.pygoogle-ads.yaml 실행 방법python google_keyword_planner.py 두 개의 파일만 있으면 구글 키워드 플래너 api 구현이 가능하다. 단,
gsixplus.tistory.com
참고 사항
- Google Ads API 접근 권한 설정: Google Ads 계정을 생성하고 Google Cloud Console에서 프로젝트를 생성 후 Google Ads API를 활성화합니다. OAuth2 클라이언트 ID와 비밀키를 생성하여 인증에 사용합니다.
- OAuth2 인증 설정: Google Ads API는 OAuth2 인증 방식을 사용합니다. Google Ads API 클라이언트 라이브러리를 활용하면 설정이 간편해집니다.
- Google Ads API 클라이언트 라이브러리 설치: 원하는 언어에 맞는 라이브러리를 설치합니다. 예를 들어, Python의 경우
google-ads
라이브러리를 사용합니다. - Keyword Planner 검색 매개변수 설정: 타겟팅, 키워드와 URL 시드 등을 설정합니다.
- API 호출로 키워드 생성: 타겟팅 매개변수를 설정한 후, GenerateKeywordIdeas 메서드를 호출하여 키워드 아이디어와 관련 메트릭을 받아옵니다.
- 키워드 데이터 활용: API 응답 데이터에는 키워드와 함께 평균 월간 검색 수, 경쟁 수준, 입찰가 등이 포함됩니다. 이러한 데이터를 바탕으로 키워드 전략을 세울 수 있습니다.
추가적으로 궁금한 점 있으면 말씀해 주세요!
'IT' 카테고리의 다른 글
authorization error: DEVELOPER TOKEN NOT APPROVED 이 (0) | 2024.10.29 |
---|---|
Google OAuth2.0 400 오류: redirect_uri_mismatch 해결방법 (0) | 2024.10.29 |
구글 키워드 애즈 API 실제로 구현 했을 때 예시 (0) | 2024.10.29 |
Google Ads Keyword Planner API 활용한 데이터 기반 블로그 콘텐츠 전략 수립과 SEO 최적화 (0) | 2024.10.29 |
Google Ads API 할당량 제한 오류 해결 가이드 (0) | 2024.10.28 |