필요한 환경
python 및 pip (Python 3.11.4)
pip install google-ads
필요한 파일
google_keyword_planner.py
google-ads.yaml
실행 방법
python google_keyword_planner.py
두 개의 파일만 있으면 구글 키워드 플래너 api 구현이 가능하다.
단, 그대로 코드를 복붙하면 안되고 특정 값은 변경해서 써야함을 주의하자!
1. 코드 (google_keyword_planner.py)
from google.ads.googleads.client import GoogleAdsClient
from google.ads.googleads.errors import GoogleAdsException
import sys
def get_keyword_ideas(customer_id, location_ids, language_id, keyword_texts):
client = GoogleAdsClient.load_from_storage("[google-ads.yaml가 위치한 경로]/google-ads.yaml")
keyword_plan_idea_service = client.get_service("KeywordPlanIdeaService")
# Create the request object
request = client.get_type("GenerateKeywordIdeasRequest")
request.customer_id = customer_id
# Set the language
request.language = f"languageConstants/{language_id}"
# Set the locations
request.geo_target_constants = [
client.get_service("GeoTargetConstantService").geo_target_constant_path(location_id)
for location_id in location_ids
]
# Set the keyword plan network
request.keyword_plan_network = client.enums.KeywordPlanNetworkEnum.GOOGLE_SEARCH_AND_PARTNERS
# Add the keyword texts
request.keyword_seed.keywords.extend(keyword_texts)
try:
# Make the request
keyword_ideas = keyword_plan_idea_service.generate_keyword_ideas(request=request)
# print(keyword_ideas)
# Process and print the results
for idea in keyword_ideas:
competition_level = client.enums.KeywordPlanCompetitionLevelEnum(
idea.keyword_idea_metrics.competition
).name
print(
f'Keyword: "{idea.text}", '
f"Avg. Monthly Searches: {idea.keyword_idea_metrics.avg_monthly_searches}, "
f"Competition: {competition_level}"
)
except GoogleAdsException as ex:
print(f"Request failed with status {ex.error.code().name} and includes the following errors:")
for error in ex.failure.errors:
print(f"\t{error.error_code}: {error.message}")
sys.exit(1)
if __name__ == "__main__":
customer_id = "[구글 애드즈에서 가입한 테스트 계정의 customer_id]"
location_ids = ["2410"] # 한국 코드
language_id = "1012" # 한국어 코드
keyword_texts = ["행복","돈","아름다움"]
get_keyword_ideas(customer_id, location_ids, language_id, keyword_texts)
* 필요시 설치사항 pip install google-ads
위 코드를 복붙하기 전에 바꿔야 될 것 두 개
- [google-ads.yaml가 위치한 경로]/google-ads.yaml
- example) "D:/googleTrendsAPI/google-ads.yaml"
- [구글 애드즈에서 가입한 테스트 계정의 customer_id]
- example) 2381517421
2. yaml파일 (google-ads.yaml)
developer_token: SdASD23SDAF323rf23fSDFSDFBC
client_id: 178978978978932-mwesdfwef22wefweeeeo3q.apps.googleusercontent.com
client_secret: SDFSDFdf-0SDFSDFDFFqQcqPDDSFSDF0TGrR-KSfDFSDFY
login_customer_id: 2381517421
use_proto_plus: True
refresh_token: 2//7e9WSDGdD_YInwA4SNwF-L9IrJmMu3kS8aASD8zrSASDD_ZwJASDSADASrEtP-CX92Jm2ASDASDSADSADls
위 YAML파일은 예시입니다.
그럼 yaml파일에 있는 각각의 토큰과 code값을 어디서 갖고 올 수 있는지 알아보겠습니다.
1. developer_token
여기에서 계정을 생성해서 개발자 토큰 발행하시면 얻을 수 있습니다.
2. client_id와 client_secret
https://console.cloud.google.com/apis/credentials
해당 페이지에서 프로젝트 생성 후 사용자 인증 정보 만들기에서 OAuth2 클라이언트 ID 생성하시면
client_id와 client_secret를 구하실 수 있습니다.
3. login_customer_id
https://ads.google.com/ 여기에서 계정을 생성해서 개발자 토큰 발행했던 것과 별개로
추가로 계정을 생성하고 해당 계정의 customer id를 갖고오면 되는데 이 것은 페이지 우측 상단에
332-191-7852 이런식으로 써있는게 있는데 이걸 쓰면 된다. 단 코드 상에서는 -를 빼고 쓰자.
4. user_proto_plus
그냥 설절 값이다 코드상 다음 이점이 있어 쓴다.
`use_proto_plus: True` 설정은 Google의 Python API 클라이언트 라이브러리(예: `google-ads`)에서 Google Protocol Buffers(프로토버프, protobuf) 메시지를 좀 더 Python 친화적으로 다룰 수 있도록 해주는 `proto-plus` 라이브러리를 활성화하는 역할을 합니다.
5. refresh_token
마지막으로 refresh_token은 해당 로그인을 매번할 수 없어 한 번 인증하고 난 후에 받는 토큰으로 api 호출시 인증할 때 로그인 대시 사용하는 토큰이다.
이 토큰은 특정 사이트에서도 받을 수 있지만 어차피 코드로 구현할 거면 해당 코드를 제공해줄테니 이 코드를 활용해서 token 발행 하시길 바란다.
generate-refresh-token.py
import json
from google.auth.transport.requests import Request
from google_auth_oauthlib.flow import InstalledAppFlow
def generate_refresh_token(client_id, client_secret):
flow = InstalledAppFlow.from_client_config(
{
"installed": {
"client_id": client_id,
"client_secret": client_secret,
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://oauth2.googleapis.com/token",
}
},
scopes=["https://www.googleapis.com/auth/adwords"],
)
# 포트를 8080으로 고정
credentials = flow.run_local_server(port=8080)
print("Access token:", credentials.token)
print("Refresh token:", credentials.refresh_token)
return credentials.refresh_token
if __name__ == "__main__":
# 여기 client_id 및 client_secret 값을 Google Cloud에서 생성한 값으로 변경
client_id = " 178978978978932-mwesdfwef22wefweeeeo3q.apps.googleusercontent.com"
client_secret: SDFSDFdf-0SDFSDFDFFqQcqPDDSFSDF0TGrR-KSfDFSDFY
refresh_token = generate_refresh_token(client_id, client_secret)
print(f"Your refresh token: {refresh_token}")
* 필요시 설치사항: pip install google-auth google-auth-oauthlib google-auth-httplib2
이전에 언급한데로 client_id와 client_secret는 예시로 적어 놓은 것이니 변경해서 넣자.
주의사항이 두 가지가 있다.
해당 코드를 python generate-refresh.token.py로 실행하면 브라우저 창에서 구글 로그인을 요청하거나 등록된 계정에서 로그인 하라고 하는데 해당 계정을 인증 받기위한 준비 단계 2개 있다.
첫 번째,
OAuth 2.0 클라이언트 ID에서 발급 받은 client_id와 client_secret의 수정 페이지로 들어가면
아래같은 칸을 볼 수 있는데 테스트 계정으로 운영하는 것이니 아래처럼 칸을 채워야한다.
(포트는 8080으로 코드 상에 넣었으니 8080으로 한다.)
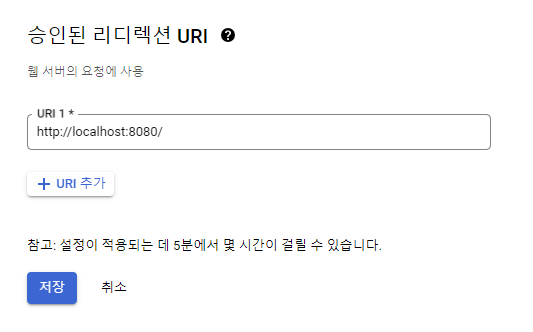
위 내용은 refresh token을 발급 신청하는 곳을 gcp에 미리 저장하여 승인 받을 수 있게하는 과정이다.
두 번째,
테스트 사용자 등록
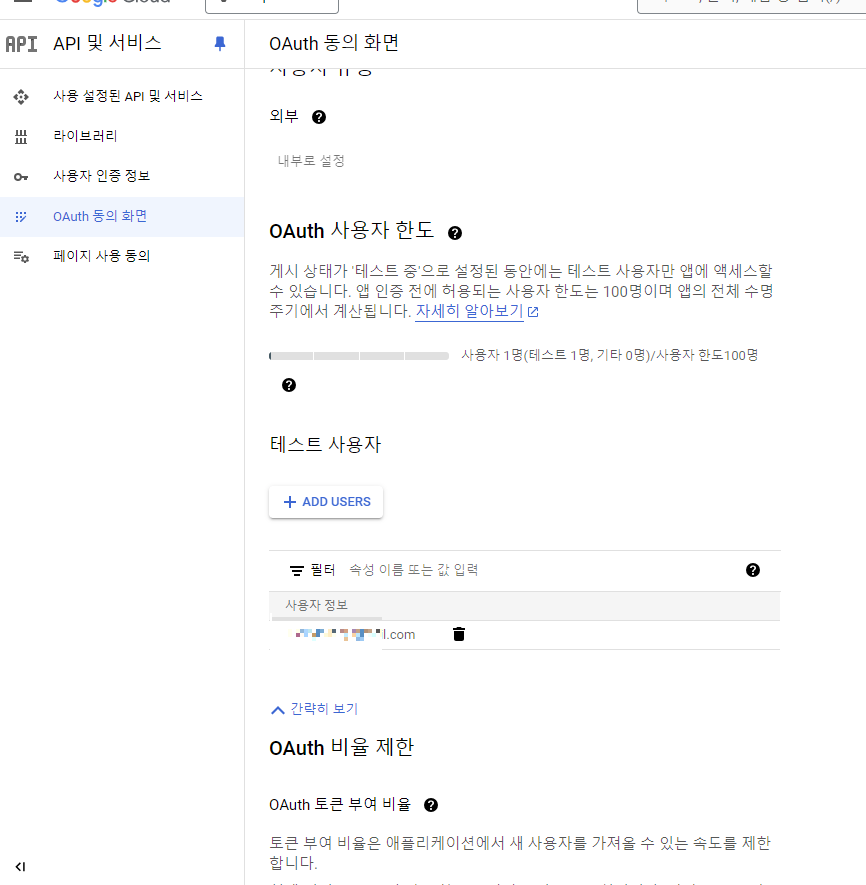
위에 내용에서 자신이 발급 받은 것을 변경해서 써야 아래처럼 정상적으로 발행이 가능하다.
python generate-refresh.token.py

위 내용을 잘 따라 했다면 다음과 같은 결과물을 콘솔 창에서 확인할 수 있다.
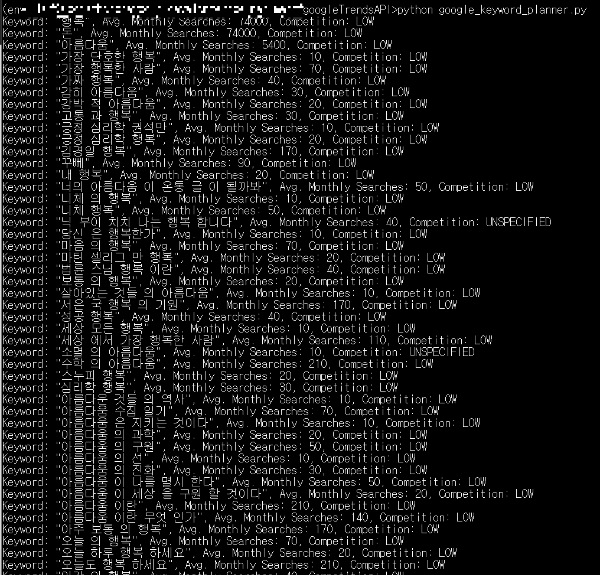
'IT' 카테고리의 다른 글
authorization error: DEVELOPER TOKEN NOT APPROVED 이 (0) | 2024.10.29 |
---|---|
Google OAuth2.0 400 오류: redirect_uri_mismatch 해결방법 (0) | 2024.10.29 |
구글 키워드 애즈 API 코드 (0) | 2024.10.29 |
Google Ads Keyword Planner API 활용한 데이터 기반 블로그 콘텐츠 전략 수립과 SEO 최적화 (0) | 2024.10.29 |
Google Ads API 할당량 제한 오류 해결 가이드 (0) | 2024.10.28 |